Synthetic sky maps¶
The LiteBIRD Simulation Framework provides the tools necessary to produce synthetic maps of the sky. These maps are useful for a number of applications:
The framework can synthetize realistic detector measurements and assemble them in Time Ordered Data (TOD).
One can run map-based simulations without the need to generate timelines: although less accurate than a full end-to-end simulation, this approach has the advantage of being much faster and requiring less resources.
The LiteBIRD Simulation Framework uses the PySM3 library to generate these sky maps; it is advisable that you keep the PySM3 manual at hand when you use the modules described in this chapter.
Here is an example showing how to use the facilities provided by the framework to generate a CMB map:
import litebird_sim as lbs
sim = lbs.Simulation(base_path="../output", random_seed=12345)
params = lbs.MbsParameters(
make_cmb=True,
fg_models=["pysm_synch_0", "pysm_freefree_1"],
)
mbs = lbs.Mbs(
simulation=sim,
parameters=params,
channel_list=[
lbs.FreqChannelInfo.from_imo(
sim.imo,
"/releases/v1.3/satellite/LFT/L1-040/channel_info",
),
],
)
(healpix_maps, file_paths) = mbs.run_all()
import healpy
healpy.mollview(healpix_maps["L1-040"][0])
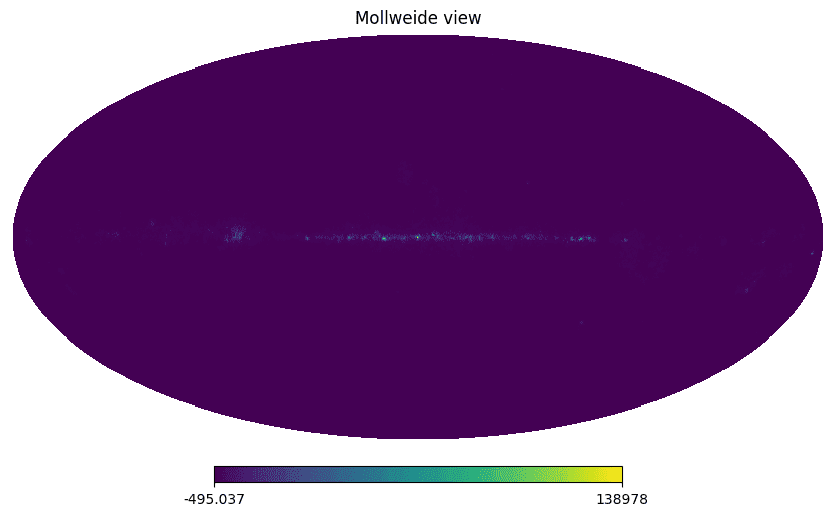
In the dictionary containing the maps Mbs returns also two useful variables:
The coordinates of the generated maps, in the key Coordinates
The parameters used for the syntetic map generation, in the key Mbs_parameters
If store_alms
in MbsParameters
is True, run_all
returns alms
instead of pixel space maps. The user can set the maximum multipole of these alms with
lmax_alms
, the default value is \(4\times N_{side}\). If gaussian_smooth
is
False, umbeamed maps or alms are returned.
Available emission models¶
The list of foreground models currently available for the
fg_models
parameter of the MbsParameters
class is the
following:
Anomalous emission:
pysm_ame_1
Dust:
pysm_dust_0
pysm_dust_1
pysm_dust_4
pysm_dust_5
pysm_dust_7
pysm_dust_8
Free-free:
pysm_freefree_1
Synchrotron:
pysm_synch_0
pysm_synch_1
Monte Carlo simulations¶
To be written!
API reference¶
- class litebird_sim.mbs.mbs.Mbs(simulation, parameters: Dict[str, Any] | str | MbsParameters = MbsParameters(nside=512, save=False, gaussian_smooth=False, bandpass_int=False, coadd=True, parallel_mc=False, make_noise=False, nmc_noise=1, seed_noise=None, n_split=False, make_cmb=True, cmb_ps_file='', cmb_r=0.0, nmc_cmb=1, seed_cmb=None, make_fg=False, fg_models=None, make_dipole=False, sun_velocity=None, output_string='date_240423', units='K_CMB', maps_in_ecliptic=False, store_alms=False, lmax_alms=2048), instrument=None, detector_list=None, channel_list=None)¶
Bases:
object
A class that generates sky maps.
This class is used to generate synthetic maps of the sky starting from the definition of a set of frequency channels and detectors.
The class uses PySM3 to generate the maps. The parameters used to set up the way sky components are generated are passed through an instance to a
MbsParameters
class, like in the following example:import litebird_sim as lbs sim = lbs.Simulation() params = lbs.MbsParameters(make_cmb=True) mbs = lbs.Mbs( simulation=sim, parameters=params, channel_list=[ lbs.FreqChannelInfo.from_imo( sim.imo, "/releases/v1.0/satellite/LFT/L1-040/channel_info", ), ], ) (healpix_maps, file_paths) = mbs.run_all()
- generate_cmb()¶
- generate_dipole()¶
- generate_fg()¶
- generate_noise()¶
- run_all()¶
Call PySM and generate the sky maps
It returns a pair
(maps, saved_maps)
, wheremaps
is a dictionary associating channels to NumPy arrays containing the maps, andsaved_maps
is a list ofMbsSavedMapInfo
objects pointing to the FITS files containing the maps.
- write_coadded_maps(saved_maps)¶
- class litebird_sim.mbs.mbs.MbsParameters(nside: int = 512, save: bool = False, gaussian_smooth: bool = False, bandpass_int: bool = False, coadd: bool | None = None, parallel_mc: bool = False, make_noise: bool = False, nmc_noise: int = 1, seed_noise: int | None = None, n_split: int | bool = False, make_cmb: bool = True, cmb_ps_file: str = '', cmb_r: float = 0.0, nmc_cmb: int = 1, seed_cmb: int | None = None, make_fg: bool = False, fg_models: Dict[str, Any] | None = None, make_dipole: bool = False, sun_velocity: list | None = None, output_string: str | None = None, units: str = 'K_CMB', maps_in_ecliptic: bool = False, store_alms: bool = False, lmax_alms: int | None = None)¶
Bases:
object
A class that specifies how sky maps should be generated by PySM3
This class is used to specify how an instance the
Mbs
class generates sky maps. You can choose to include the following components in the maps:CMB signal (use
make_cmb=True
);Foreground signal (use
make_fg=True
);Solar dipole signal (use
make_dipole=True
)Noise (use
make_noise=True
); this should be used only when running map-based simulations, because if you are creating time-ordered data, chances are that you want to inject noise directly in the timelines.
The full list of parameters and their default values are listed here:
nside
(default: 512): value of theNSIDE
parameter to use when creating the mapssave
(default:False
): whenTrue
, Healpix maps are saved as FITS files in the output path (specified by aSimulation
object)gaussian_smooth
(default:False
): whenTrue
, maps are smoothed using a Gaussian beam whose width is the FWHM of the frequency channel.bandpass_int
(default:False
): whenTrue
, maps are integrated over the bandpass of each detectorcoadd
(default:None
): whenTrue
, maps are coaddedparallel_mc
(default:False
): whenTrue
, Monte Carlo realizations are computed in parallel using MPImake_noise
(default:False
): whenTrue
, noise maps are generated and added to the final result;nmc_noise
(default: 1): number of Monte Carlo runs for noise mapsseed_noise
(default:None
): when specified, it must be a positive integer number used as the seed of the pseudo-random number generatorn_split
(default:False
): when specified as an integer, number of splits used to generate noise mapsmake_cmb
(default:True
): whenTrue
, a CMB map is generated and added to the final resultcmb_ps_file
(default:""
): if specified, it must be the path to a FITS file containing the CMB power spectrum to be used to generate the CMB mapcmb_r
(default: 0.0): value of the tensor-to-scalar ratio to be used when generating the CMB mapnmc_cmb
(default: 1): number of Monte Carlo realizations for CMB mapsseed_cmb
(default: None): if specified as an integer, the seed used to initialize the random number generator used to create a CMB realizationmake_fg` (default: ``False
): whenTrue
, a foreground map is generated and added to the mapsfg_models
(default:None
): this specifies the foreground models as a list of strings, each specifying a foreground component. Alternatively, it can be provided as a dictionary which associates a name to a component, e.g.,{"ame": "pysm_ame_1"}
.make_dipole` (default: ``False
): whenTrue
, a solar dipole map is added to the mapssun_velocity
(default:None
): this list specifies the direction, latitude (rad) and longitude (rad) in galactic coordinates, and the amplitude in Km/s of the dipole.IfNone
these values are taken .constants. The dipole implemented is the “Linear approximation in β using thermodynamic units” as in :class:.dipole.DipoleType
.output_string
(default:""
): a string used to build the file names of the Healpix FITS files saved by theMbs
class.units
(default:K_CMB
): string used to specify the measurement unit of the pixels in the maps generated by theMbs
class. It follows the naming of the pysm3 units, e.g. “K_CMB” / “K_RJ” / “uK_CMB” / “uK_RJ”maps_in_ecliptic
(default:False
): whenTrue
the maps contained in the dictionary returned by Mbs.run_all are converted in ecliptic coordinates using the healpy routine rotate_map_almsstore_alms
(default:False
): whenTrue
the maps contained in the dictionary returned by Mbs.run_all are stored as alms computed by the healpy routine map2alm assuming iter=0 If you want unbeamed alms set gaussian_smooth = Falselmax_alms
(defuaul:4 x nside
): lmax assumed in the alm computation and in the rotation to ecliptic coordinates performed by rotate_map_alms
- bandpass_int: bool = False¶
- cmb_ps_file: str = ''¶
- cmb_r: float = 0.0¶
- coadd: bool | None = None¶
- fg_models: Dict[str, Any] | None = None¶
- static from_dict(dictionary)¶
Create a
MbsParameters
instance from a dictionaryTypically, the dictionary passed to this static method should be taken from a TOML file, possibly through the param field of a
Simulation
object, like in the following example:import litebird_sim as lbs sim = lbs.Simulation(parameter_file="config.toml") mbs_params = lbs.MbsParameters.from_dict(sim.params.["mbs_params"])
- gaussian_smooth: bool = False¶
- lmax_alms: int | None = None¶
- make_cmb: bool = True¶
- make_dipole: bool = False¶
- make_fg: bool = False¶
- make_noise: bool = False¶
- maps_in_ecliptic: bool = False¶
- n_split: int | bool = False¶
- nmc_cmb: int = 1¶
- nmc_noise: int = 1¶
- nside: int = 512¶
- output_string: str | None = None¶
- parallel_mc: bool = False¶
- save: bool = False¶
- seed_cmb: int | None = None¶
- seed_noise: int | None = None¶
- store_alms: bool = False¶
- sun_velocity: list | None = None¶
- units: str = 'K_CMB'¶
- class litebird_sim.mbs.mbs.MbsSavedMapInfo(path: Path | None = None, component: str = '', channel: str | None = None, mc_num: int | None = None)¶
Bases:
object
Information about a map saved by the
Mbs
classInstances of this class are usually returned by the method
Mbs.run_all()
. They have the following fields:path
: a pathlib.Path object representing the Healpix FITS map saved on diskcomponent
: a string identifying the component; possible values arecmb
,fg
,noise
, andcoadded
.channel
: the name of the frequency channel (it isNone
for CMB maps)mc_num
: it can either beNone
or the number of a Monte Carlo realization
- channel: str | None = None¶
- component: str = ''¶
- mc_num: int | None = None¶
- path: Path | None = None¶